PHP Model Test Questions and Answers
1. Define Heredocs and Nowdocs in PHP?
Nowdocs and Heredocs are ideal for embedding PHP code or other large blocks of text without the need for escaping.
Heredoc parse PHP variables and html elements within its block.
$foo = 'here';
$here = <<<HERE
I'm new $foo!
HERE;
Output: I'm new here!
Nowdoc can not parse PHP variables and html elements within its block it prints just only as a plain text. For Nowdoc quoted is required in nowdoc keyword.
$foo='here';
$now = <<<'NOW'
I'm new $foo!
NOW;
Output: I'm new $foo!
2. What are constructor and destructor?
Answer: Constructor: a constructor is a special function that gets called automatically when the object of a class is created. A constructor will have exact same name as the class or __construct() name and it does not have any return type at all, not even void. Constructors can be very useful for setting initial values for certain member variables.
Destructor: Destructor is a special function that gets called automatically when a class object is deleted or goes out of scope.
3. What are super global variables? List all super global variables?
The super global variables are predefined variable in PHP. These are accessible from anywhere within a substantial amount of environment specified information.
Name of some super global variable:-
$_GET[], $_POST[], $_REQUEST[], $_SERVER[], $_SESSION[], $GLOBAL[], $_COOKE[], $_FILES[] and $_ENV[].
4. What is property and method overloading in PHP?
Overloading traditionally provides the ability to have multiple methods with the same name but different quantities and types of arguments.
But in PHP Properties and methods are those entities created dynamically by using PHP appropriate magic methods: __set(),__get() etc. After creating object for a class, we can access set of entities, that are, properties or methods not defined within the scope of the class. Such entities are said to be overloaded properties or methods, and the process is called as overloading.
5. What are the abstract class and interface?
Abstract class: An Abstract class is a class that cannot be instantiated but instead serves as a base class to be inherited by other classes.
Interface: An Interface is a collection of unimplemented method definitions and constants without specifying exactly how it must be implemented.
6. Difference between array_merge() and array_slice()?
Answer: The array_merge() function merges one or more arrays into one array. This function returns one array with the elements of all the parameter arrays.
The array_slice() function returns selected parts of an array. The array_slice() function returns part of an array as specified by the start and length parameters. If start is a positive number, it means that the ”slice” will start that many elements from the beginning of the array.
7. What are classes and objects?
Answer: Class: Classes are intended to represent those real-life items that coder like to manipulate within an application.
object: An instance of class is called object. Once the object is created, all characteristic and behaviors define within the class are made available to the newly instantiated object.
8. What are type casting and juggling?
Casting: Converting values from one data type to another is known as type casting. This is accomplished by placing the intended type in front of the variable to be cast.
example:
$x=34.54;
$y=(int)$x;
echo $y; // returns 34
Juggling: Variables are sometimes automatically cast to best fit the circumstances in which they are referenced. This is known as Type Juggling.
example:
$a="34 items"
$total=5;
$total+=$a;
echo $total;// returns 39
9. Define exceptions?
Answer: An exception is an abnormal condition that arises in a code sequence at run time. Basically there are four important keywords which form the main pillars of exception handling: try, catch, throw and finally.
10. What are the differences between public, private, protected, static, transient, final and volatile?
Answer:
Public: Public declared items can be accessed everywhere.
Protected: Protected limits access to inherited and parent classes (and to the class that defines the item).
Private: Private limits visibility only to the class that defines the item.
Static:
Static Variable: A static variable exists only in a local function scope, but it does not lose its value when program execution leaves this scope. Static Function: static function can be access without create class object.
Final: Final keyword prevents child classes from overriding a method by prefixing the definition with final. It means if we define a method with final then it prevent us to override
the method.
transient: A transient variable is a variable that may not be serialized.
volatile: A variable that might be concurrently modified by multiple threads should be declared volatile. Variables declared to be volatile will not be optimized by the compiler because their value can change at any time
11. What are the four configuration directive scopes?
PHP_INI_PERDIR: Directive can be modified within the php.ini, httpd.conf, or .htaccess files.
PHP_INI_SYSTEM: Directive can be modified within the php.ini and httpd.conf files.
PHP_INI_USER: Directive can be modified within user scripts.
PHP_INI_ALL: Directive can be modified anywhere.
12. What are the advantages of object-oriented programming?
a. Simplicity
b. Modifiability
c. Re-usability.
d. Extensibility
e. Catch errors at compile time rather than at runtime
f. Reduce large problems to smaller
13. Discuss the following PHP array functions:
array_reverse(): reverses an array’s element order.
array_flip(): reverses the roles of the keys and their corresponding values.
sort(): sorts an array, ordering elements from lowest to highest value.
rsort(): sorts array items in reverse (descending) order.
asort() : sorting an array in ascending order, except that the key/value correspondence is maintained.
arsort(): it sorts the array in reverse order maintained key/value co-relation.
14. What is recursive function?
A recursive function is a function that calls itself during its execution. This enables the function to repeat itself several times, outputting the result and the end of each iteration. Below is an example of a recursive function.
15. What is constant? How can you declare a constant in procedural method?
A constant is a value that cannot be modified throughout the execution of a program. Constant are declare by using the define() function. Its prototype follows:
boolean define(string name, mixed value)
Example
define("PI",3.14159);
echo PI; //returns 3.14159
16. What is x+ mode in fopen() used for?
Answer: Read/Write. Creates a new file. Returns FALSE and an error if file already exists
17. What are the different types of errors in PHP?
i. Notices: These are trivial, non-critical errors that PHP encounters while executing a script - for example,
accessing a variable that has not yet been defined. By default, such errors are not displayed to the user at all
- although you can change this default behavior.
ii. Warnings: These are more serious errors - for example, attempting to include() a file which does not exist.
By default, these errors are displayed to the user, but they do not result in script termination.
iii. Fatal errors: These are critical errors - for example, instantiating an object of a non-existent class, or calling
a non-existent function. These errors cause the immediate termination of the script, and PHP’s default
behavior is to display them to the user when they take place.
18. Write four general language features of php?
Answer:
Practicality: It requires minimum knowledge of programming.
Power: It has the ability to interface with databases, form and create pages dynamically.
Possibility: Developers are rarely bound to any single implementation solution.
Price: It is open source software.
19. What are passing arguments by reference and passing arguments by value?
Passing arguments by value: If the value of the argument within the function is changed, it does not get changed outside of the function.
Passing arguments by reference: To allow a function to modify its arguments, they must be passed by reference. It prepends an ampersand (&) to the argument name in the function definition
20. What are optional or default arguments? How can you define optional argument in PHP?
A default argument or optional argument is an argument to a function that a programmer is not required to specify.
function add($a,$b,$c=0){
echo $a+$b+$c;
}
//here $c is option/default argument
add(3,4);//we are not specifying default argument here.
add(3,4,5);
21. Write down the five associative keys of $_FILES[] super global.
22. Define scope resolution operator (::) operator
It is useful to refer to functions and variables in base classes or to refer to functions in classes that have not yet any instances. The :: operator is being used for this.
23. Discuss the keywords break,return and continue
24. What do you mean by control structure?
Following the structured program theorem, all programs are seen as composed of control structures:
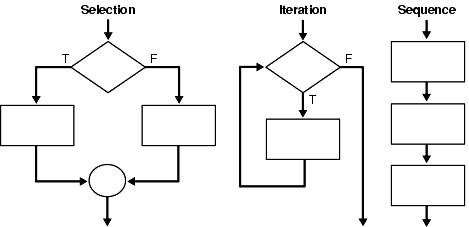
1. "Sequence"; ordered statements or subroutines executed in sequence.
2. "Selection"; one or a number of statements is executed depending on the state of the program. This is usually expressed with keywords such as if..then..else..endif.
3. "Iteration"; a statement or block is executed until the program reaches a certain state, or operations have been applied to every element of a collection. This is usually expressed with keywords such as while, repeat, for or do..until. Often it is recommended that each loop should only have one entry point (and in the original structural programming, also only one exit point, and a few languages enforce this).
4. "Recursion"; a statement is executed by repeatedly calling itself until termination conditions are met. While similar in practice to iterative loops, recursive loops may be more computationally efficient, and are implemented differently as a cascading stack.
PHP MCQ TEST
1. Which is the single-line comments C++ syntax in php?
A) //
B) --
C) <-->
D) <??>
2. Which ASP-Style tag support php?
A) <% %>
B) </ />
C) <- ->
D) <: :>
3. Which are outputting functions?
A) write()
B) print()
C) print_r()
D) echo
4. Which can be able to automatically destroy object?
A) constructor
B) destructor
C) class
D) object
5. What do you mean by function prototype?
A) Simply the function's definition
B) Function’s Input parameters.
C) defined data type
D) Function’s returns type.
6. PHP_INI_PERDIR: Directive can be modified within the php.ini, httpd.conf files.
A) True
B) False
7. Which indicates a sequence of characters that treated as a contagious group?
A) String
B) array
C) printf
D) integer
8. Which allows you to specify numbers that contains fractional parts?
A) Float
B) String
C) array
D) Boolean
9. Which of the followings are variable scope?
A) Local variable
B) Global variable
C) Static variable
D) Functions parameters
E) All
10. Which statements match against identifiers?
A) It’s case sensitive
B) It can contain data
C) Starts with a $ sign
D) Variable name can only contain A-z, 0-9, and _
E) C and B
11. What will be the correct output of the following syntax?
1. <?php
2. $total=”45 fire engines”;
3. $incoming=”10”;
4. $total=$incoming+$total
5. ?>
A) 55
B) 45 fire engines
C) 45 fire engines10
D) 55 fire engines
12. Which indicates a general term that we can apply to variables,functions and various other user defined objects?
A) Identifiers
B) Compound data type
C) Array
D) Symbol
13. Which is identified as a special variable, which can store multiple values in one single variable?
A) Array
B) Variable
C) Functions
D) Operator
14. function my_function(){
static $count=1;
$count++;
echo $count;
echo "<br/>";
}
my_function();
my_function();
my_function();
What will be the correct out of the above static syntax?
A) 2 3 4
B) 1 2 3
C) 1 0 1
D) error
15. Which function returns the type of variable?
A) gettype()
B) settype()
C) ini_set()
D) none of the above
16. For adding a value at the first index position of an array what we use?
A) The array_unshift() function
B) The array_shift() function
C) The array_push() function
D) The array_pop() function
17. What is the output of the following array function?
$a = range(0, 20, 2);
A) $a = array(0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20);
B) $a = array( 2, 4, 6, 8, 10, 12, 14, 16, 18, 20);
C) $a = array(2, 4, 6, 8, 10, 12, 14, 16, 18);
D) None
18. The in_array() function _______
A) search an array for a specific value.
B) push an array for a specific value.
C) shift an array for a specific value.
D) none
19. $states = array("Florida");
$state = "Ohio";
printf("$states is an array: %s <br />", (is_array($states) ? "TRUE" : "FALSE"));
A) $state is an array: FALSE
B) $states is an array: TRUE
C) $states is an array: NULL
D) None
20. What is the output of the following script?
$states = array("Ohio", "New York");
array_unshift($states, "Arizona", "Texas");
print_r($states)
A) Array ( [0] => Arizona [1] => Texas [2] => Ohio [3] => New York )
B) array ( [0] => Arizona [1] => Texas [2] => Ohio [3] => New York )
C) Array ( [0] => Ohio [1] => New York [2] => Arizona [3] => Texas )
D) $states=array("Arizona", "Texas", "Ohio", "New York");
21. $state["Delaware"] = "December 7, 1787";
$state["Pennsylvania"] = "December 12, 1787";
$state["New Jersey"] = "December 18, 1787";
$keys = array_keys($state);
print_r($keys);
What will be the output?
A) Array ( [0] => Delaware [1] => Pennsylvania [2] => New Jersey )
B) Array ( “Delaware”,” Pennsylvania” ,”New Jersey” )
C) array ( [0] => Delaware [1] => Pennsylvania [2] => New Jersey )
D) Array ( [0] => Delaware ,[1] => Pennsylvania ,[2] => New Jersey )
22. Which of the followng is the correct session related directive to enable session throughout the site?
A) session_auto_start=1;
B) session.auto_start=1
C) session.start=1
D) session.autostart=1
23. What is the output for the following code?
<?php
$total=0;
for($i=0;$i<4;$i++){
$total=$total+$i;
}
echo $total;
?>
A) 6
B) 4
C) 5
24. Which of the following PHP function is used to send email?
A) email()
B) mail()
C) send_mail()
D) send_email()
25. What is the following is the correct command for installing PEAR mail and mime package?
A) %>pear install Mail Mail_Mime
B) %>pear setup Mail Mail_Mime
C) %>pear setup mail mail_mime
D) %>pear install mail mail_mime
26. SimplePie is a ______
A) RSS perser
B) API
C) XML perser
D) Extension for RSS
27. SimpleXML is a _____
A) Methodology for parsing XML.
B) API
C) feed
D) XML language
28. Which of the following PHP function is used to determine the length of any string?
A) strlen()
B) str_len()
C) count()
D) length()
29. To store a complete hash in a database, you need to set the field length to _____ for md5()
A) 32
B) 16
C) 28
D) 50
30. implode function is used to _____
A) concatenate array elements to form a string
B) concatenate string elements to form a string
C) split string into and array
D) none
31. Which of the following function is used to divides the string into an array of sub strings using delimiter?
A) explode()
B) substr()
C) split_str()
D) sub_str()
32. Which of the following PHP function is used to clear HTML tags from sting?
A) remove_tags()
B) strip_tags()
C) clear_tags()
D) cleartags()
33. htmlspecialchars() is used to convert special characters into HTML entities and they are:
A) & " ' < >
B) & " ' < > #
C) & " ' < > # _
D) & " ' < > _ # *
34. Which symbol is used for reference assignment?
A) $
B) &
C) @
D) ;
35. What is the output of the following:
1. $str= "good";
2. $$str= "bye";
3. echo ${$str};
A) good
B) bye
C) good bye
D) None
36. What is the output of the following code?
1. $a=1;
2. ++$a;
3. $a*=$a;
4. echo $a--;
A) 3
B) 4
C) 5
D) 0
E) 1
37. Write the output of echo ("35 hello 55"+65); statement?
A) 155
B) 120
C) 100
D) 90
38. What function is used manipulating PHP configuration variable?
A) unset()
B) ini_set()
C) php_conf
D) None
39. A ______ is a value that cannot be modified throughout the execution of a program.
A) Constants
B) Variables
C) Expressions
D) None
40. <?php
$title = "O'Malley wins the heavyweight championship!";
echo ucwords($title);
?>
Which of the following is correct output for above Statement ?
A) O'Malley wins the heavyweight championship!
B) O'Malley Wins The Heavyweight Championship!
C) O'Malley wins the Heavyweight Championship!
D) O'MALLEY WINS THE HEAVYWEIGHT CHAMPIONSHIP !
41. Which of the following is not a part of OOP?
A) Type checking
B) Inheritance
C) Polymorphism
D) Encapsulation
42. A constructor is used to ____
A) Free memory.
B) Initialize a newly created object.
C) Import packages.
D) Create a JVM for applets.
43. Abstract class objects never have to be instantiated.
A) True
B) False
44. Which of the following function returns the number of characters in a string variable?
A) count($variable)
B) len($variable)
C) strcount($variable)
D) strlen($variable)
45. Which quantifiers matches any string containing a p followed by zero or more instances of the sequence hp ?
A) p(hp)*
B) p*(hp)
C) *hp(p)
D) hp(p)*
46. Which quantifiers matches any string with p at the beginning of it?
A) p+
B) p?
C) ^p
D) p^
47. <?php
$grades = array(42, "hello", 42);
$total = array_sum($grades);
print $total;
?>
Which will print?
A) 48
B) 84
C) 44
D) 42 hello 42
48. Which is/are the OOP's foundational concept?
A) encapsulation
B) inheritance
C) polymorphism
D) all
49. How to convert a string into lowercase?
A) Stringtolower($str)
B) tolower($str)
C) strtolower($str)
D) lower($str)
50. We can get the client’s IP address by
A) $_SERVER['REMOTE_ADDR']
B) $_SERVER['REMOTE_addr']
C) $_SERVER$_REMOTE_ADDR
D) $_SERVER(){'REMOTE_ADDR'}
Comments 1