Example of React JSON data with fetch() function
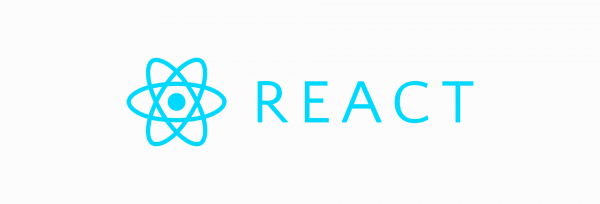
import React, { Component } from 'react'
export class Subject extends Component {
constructor(props){
super(props);
this.state={items:[],error: null,isLoaded: false};
}
componentDidMount(){
this.loadJSON();
}
loadJSON(){
fetch("/android/menu_by_grade_json.php?grade=2")
.then(res => res.json())
.then(
(result) => {
console.log(result.subject);
this.setState({
isLoaded: true,
items: result.subject
});
},
(error) => {
this.setState({
isLoaded: true,
error
});
}
)
}
render() {
return (
<div>
<ul>
{this.state.items.map(item => (
<li key={item.id}>
{item.name}
</li>
))}
</ul>
</div>
)
}
}
export default Subject
Example of nested map() function
render() {
if(this.state.isLoaded){
return (
<div>
<ul>
{this.state.subjects.map(item => (
<li key={item.id}>
{item.id} {item.name}
<ul>
{
item.chapter.map(chap=>{
return <li key={chap.id}>{chap.name}</li>
})
}
</ul>
</li>
))}
</ul>
</div>
)
}else{
return (
<div>
Subjects not found
</div>
)
}
}
Comments 0