React and Larave 8.x API
Note: Assuming that you have a Laravel App as the following path:
c:xampphtdocslaravelapp1
To create new Laravel app go to (c:xampphtdocslaravel) folder
with command prompt and write the following command as:
c:xampphtdocslaravel>composer create-project laravel/laravel app1
To get into the project folder enter the following command:
c:xampphtdocslaravel>cd app1
Also assuming that you also have product and category tables in your system as follows:
DROP TABLE IF EXISTS `test`.`core_categories`;
CREATE TABLE `test`.`core_categories` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(45) NOT NULL,
`section_id` int(10) unsigned NOT NULL,
`created_at` timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),
`updated_at` datetime DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
DROP TABLE IF EXISTS `test`.`core_products`;
CREATE TABLE `test`.`core_products` (
`id` int(10) NOT NULL AUTO_INCREMENT,
`name` varchar(50) DEFAULT NULL,
`offer_price` double DEFAULT NULL,
`manufacturer_id` int(10) DEFAULT NULL,
`regular_price` double NOT NULL,
`description` text DEFAULT NULL,
`photo` varchar(50) DEFAULT NULL,
`category_id` int(10) unsigned NOT NULL,
`section_id` int(10) unsigned NOT NULL,
`is_featured` tinyint(1) NOT NULL DEFAULT 0,
`star` int(10) unsigned NOT NULL,
`is_brand` tinyint(1) NOT NULL DEFAULT 0,
`offer_discount` float NOT NULL DEFAULT 0,
`uom_id` int(10) unsigned NOT NULL,
`weight` float NOT NULL,
`barcode` varchar(45) NOT NULL,
`created_at` datetime DEFAULT current_timestamp(),
`updated_at` datetime DEFAULT current_timestamp(),
PRIMARY KEY (`id`),
UNIQUE KEY `uni_barcode` (`barcode`),
UNIQUE KEY `uni_name` (`name`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
You also need all necessary database connectivity settings with laravel. See the laravel documentation
Step 1: Open your app1 using Visual Studio Code.
Step 2: Create a folder named API inside the AppHttpController folder
AppHttpControllerAPI
Step 3: Load laravel project app1 with Visual Studio Code applying cmd command
c:>cd c:xampphtdocslaravelapp1
c:xampphtdocslaravelapp1>code .
(Visual Studio Code editor will be launch with your project)
Step 4: Create Models : Category , Product as the following artisan command:
c:xampphtdocslaravelapp1>php artisan make:model Category
c:xampphtdocslaravelapp1>php artisan make:model Product
Step 5: Create Controllers to API folder: CategoryController, ProductController as the following command:
c:xampphtdocslaravelapp1>php artisan make:controller API/CategoryController --api
c:xampphtdocslaravelapp1>php artisan make:controller API/ProductController --api
Step 6: Open up
api.php located at app/routes folder
and add the following: lines:
Route::apiResources([
'products' => ProductController::class,
'categories' => CategoryController::class,
]);
then save the file
Step 7: Open your ProductController.php from AppHttpControllerAPI
and write the following code:
<?php
namespace AppHttpControllersAPI;
use AppModelsProduct;
use AppHttpControllersController;
use IlluminateHttpRequest;
class ProductController extends Controller
{
/**
* Display a listing of the resource.
*
* @return IlluminateHttpResponse
*/
public function index()
{
return response()->json(["products"=>Product::All()]);
}
/**
* Store a newly created resource in storage.
*
* @param IlluminateHttpRequest $request
* @return IlluminateHttpResponse
*/
public function store(Request $request)
{
$product=new Product;
$product->name=$request->txtName;
$product->offer_price=$request->txtOffer_price;
$product->manufacturer_id=$request->cmbManufacturerId;
$product->regular_price=$request->txtRegular_price;
$product->description=$request->txtDescription;
if(isset($request->filePhoto)){
$product->photo=$request->filePhoto;
}
$product->category_id=$request->cmbCategoryId;
$product->section_id=$request->cmbSectionId;
$product->is_featured=$request->txtIs_featured;
$product->star=$request->txtStar;
$product->is_brand=$request->txtIs_brand;
$product->offer_discount=$request->txtOffer_discount;
$product->uom_id=$request->cmbUoMId;
$product->weight=$request->txtWeight;
$product->barcode=$request->txtBarcode;
$product->save();
if(isset($request->filePhoto)){
$imageName = $product->id.'.'.$request->filePhoto->extension();
$product->photo=$imageName;
$product->update();
$request->filePhoto->move(public_path('img'),$imageName);
}
//return response()->json(['success'=>'Saved']);
return json_encode(['success'=>'Saved']);
}
/**
* Display the specified resource.
*
* @param int $id
* @return IlluminateHttpResponse
*/
public function show($id) {
return json_encode(Product::find($id));
}
/**
* Update the specified resource in storage.
*
* @param IlluminateHttpRequest $request
* @param int $id
* @return IlluminateHttpResponse
*/
public function update(Request $request,$id)
{
$product=Product::find($id);
$product->name=$request->txtName;
$product->offer_price=$request->txtOffer_price;
$product->manufacturer_id=$request->cmbManufacturerId;
$product->regular_price=$request->txtRegular_price;
$product->description=$request->txtDescription;
if(isset($request->filePhoto)){
$product->photo=$request->filePhoto;
}
$product->category_id=$request->cmbCategoryId;
$product->section_id=$request->cmbSectionId;
$product->is_featured=$request->txtIs_featured;
$product->star=$request->txtStar;
$product->is_brand=$request->txtIs_brand;
$product->offer_discount=$request->txtOffer_discount;
$product->uom_id=$request->cmbUoMId;
$product->weight=$request->txtWeight;
$product->barcode=$request->txtBarcode;
if(isset($request->filePhoto)){
$imageName = $product->id.'.'.$request->filePhoto->extension();
$product->photo=$imageName;
$request->filePhoto->move(public_path('img'),$imageName);
}
$product->update();
return json_encode(["success"=>$id]);
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return IlluminateHttpResponse
*/
public function destroy($id)
{
Product::find($id)->delete();
return json_encode(["success"=>$id]);
}
}
Same way you write code for CategoryController
Step 8:
Download and setup Postman software.
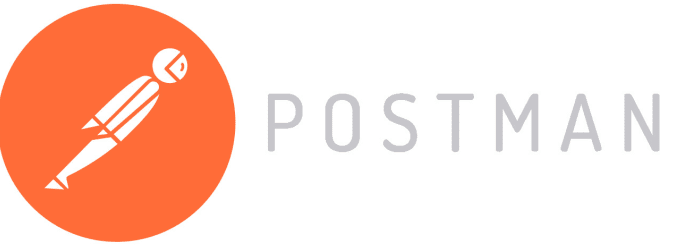
Now check and verify if your laravel api is working fine or not using Postman.
Lunch your Postman software
Add new tab on clicking + button
check all the following endpoints one by one:
For product table:
1. To see all records:
localhostlaravelapp1publicapiproducts using GET method
2. To see a record:
localhostlaravelapp1publicapiproducts1 using GET method
3. To delete a record:
localhostlaravelapp1publicapiproducts1 using DELETE method
4. To insert a record:
localhostlaravelapp1publicapiproducts using POST method
select body radio > raw radio button below the url then select JSON
and add your required testing key: value data (No id key is required) as follows:
{
"txtName":"Apple",
"txtOffer_price":300,
"cmbManufacturerId":1
...
}
5. To update a record:
localhostlaravelapp1publicapiproducts1 using PUT method
select body radio > raw radio button below the url then select JSON
and add your required testing key: value data as follows:
{
"txtName":"Orange",
"txtOffer_price":300,
"cmbManufacturerId":1
...
}
Comments 0